Introducing C++ For loop, Using Manipulators and revision of concept of Class and Object
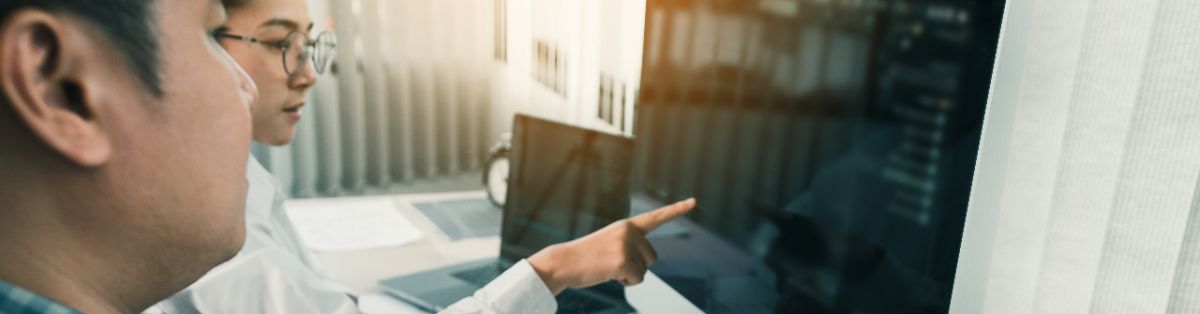
Lab Session on July 31, 2023:
Sam: Hey Alex, that was quite an intense lab session today! We covered so many topics.
Alex: Yeah, it was really comprehensive. The for loop, classes and objects, file handling, and even manipulators. I feel like we learned a lot!
Compy: It's always great to build on the foundations. Why don't we start with the basics? How about we discuss the for loop first?
Sam: Sounds like a plan! I think I've got a grasp of it, but a little review couldn't hurt.
Introduction to the for Loop
Compy: The for loop is a control structure that allows a block of code to be executed multiple times. Here's a simple syntax:
for(initialization; condition; update) { // Code to be executed }
Alex: Right, and the initialization is where we set up the loop variable, the condition is what keeps the loop running, and the update changes the loop variable each time through the loop.
Sam: So it's like a while loop, but everything is in one line at the top!
Compy: Exactly, it's a very compact way to repeat code. Shall we move on to iterating over a string with a for loop?
Iterating over a String with a for Loop
Compy: Iterating over a string with a for loop is a common task in programming. Let's say we have a string and we want to print each character separately. Here's how we could do it:
std::string str = "VIT University"; for(int i = 0; i < str.length(); i++) { std::cout << str[i] << ' '; }
Alex: So the loop runs from 0 to the length of the string minus one, and we use the loop variable i
as an index to access each character. Simple!
Sam: Exactly! It's like treating the string as an array of characters.
Compy: That's a perfect way to describe it. This approach can be used for various operations on strings, such as reversing, counting specific characters, and more. Shall we move on to revising the concepts of classes and objects?
Revision of Class and Object Concepts
Sam: Oh, I'm eager to revisit classes and objects. I feel like I need a refresher!
Compy: Classes and objects are central to object-oriented programming (OOP). A class is a blueprint that defines the structure and behavior of objects. Objects are instances of classes. Here's a simple example:
class Student { public: std::string name; int age; Student(std::string n, int a) { name = n; age = a; } void display() { std::cout << "Name: " << name << " Age: " << age; } }; Student s1("Alex", 20); s1.display(); // Outputs: Name: Alex Age: 20
Alex: So a class defines the structure, and we can create multiple objects using that class, each with different data.
Sam: Exactly! It's like creating different student profiles using the same blueprint.
Compy: Here is a complete example that you can run. Observe the similarities. Without main()
, the previous snippet would not run.
#include <iostream> #include <string> class Person { std::string name; public: Person(std::string name) { this->name = name; } void introduce() { std::cout << "Hello, my name is " << name << "\n"; } }; int main() { Person p1("Alex"); p1.introduce(); // Output: Hello, my name is Alex return 0; }
Using for Loop to Print Multiplication Table
Compy: The for loop is highly useful in generating patterns and sequences, like a multiplication table. Shall we print a multiplication table for a given number?
Sam: That sounds interesting. Let's do it for the number 5!
for(int i = 1; i <= 10; i++) { std::cout << "5 x " << i << " = " << 5 * i << std::endl; }
Alex: I see how it works. The loop iterates from 1 to 10, and for each iteration, it prints the multiplication of 5 with the current value of i
.
Making the Output Neat with Manipulators
Compy: Manipulators are great for formatting the output neatly. Here's how we can use setw
and setfill
to align the multiplication table:
#include <iomanip> // ... for(int i = 1; i <= 10; i++) { std::cout << std::setw(2) << "5 x " << std::setw(2) << i << " = " << std::setw(2) << 5 * i << std::endl; }
Alex: Wow, it looks much neater now! The numbers are aligned perfectly.
Sam: I can see how this could be useful in formatting bills or any other structured data. What's next, Compy?
Compy: Before we go further, why don't you try to complete the code snippet on your own so that it executes without any error? Did you know, you can use the similar concepts to write to the file system also?
Casual Discussion with Sam, Alex, and Compy
Sam: Today's lab session was intense, wasn't it, Alex? We covered so much ground!
Alex: Absolutely, Sam. I found the part about the file handling class particularly interesting. It made me realize how classes can be used to organize complex functionality into manageable parts.
Compy: That's the power of object-oriented programming! By encapsulating related functionality within classes, you create more maintainable and reusable code. How did you feel about the for loop and manipulators part?
Sam: The for loop was pretty straightforward, but I was amazed at how manipulators like setw
and setfill
can make the output look so neat. It's like having control over the design without needing any special tools.
Alex: Right, and the use of tellp
in file handling brought home the point that there's a lot more to files than just reading and writing. There are various nuances and techniques to master.
Compy: Exactly, Alex. Combining these concepts, you can now create more sophisticated programs. Remember, practice makes perfect, so keep experimenting with what you've learned.
Sam: We will, Compy. Thanks for helping us reflect on today's topics. It's always great to have these discussions with you and Alex.
Alex: Agreed, Sam. Compy, your insights are invaluable. Looking forward to our next session!
Compy: Thank you, both. Keep exploring, learning, and having fun with programming. See you next time!
Compendium of Terms
- Flash Memory
- A type of non-volatile storage that can be electrically erased and reprogrammed.
- HDD (Hard Disk Drive)
- A data storage device that uses magnetic storage to store and retrieve digital information.
- SSD (Solid State Drive)
- A storage device that uses integrated circuit assemblies to store data persistently.
- P/E Cycles (Program/Erase Cycles)
- The finite number of program/erase cycles an SSD cell can endure before becoming unreliable.
- FAT (File Allocation Table)
- An older file system designed for small disks and simple folder structures.
- NTFS (New Technology File System)
- A file system introduced by Microsoft with improvements over FAT, such as support for larger files and volumes, and enhanced security features.
- Sequential Access
- Reading data in a sequence, from beginning to end.
- Random Access
- The ability to access any part of a file without reading the parts before it.
- Stream
- In C++, a general term for a sequence of bytes for input or output.
- File Stream
- A specific type of stream in C++ used for file input and output.
- cin
- A C++ stream object used to read input from the keyboard.
- cout
- A C++ stream object used to output data to the screen.
- cerr
- A C++ stream object used for outputting unbuffered error messages.
- clog
- A C++ stream object used for outputting buffered error messages.
- ifstream
- A file stream object in C++ for reading data from files.
- ofstream
- A file stream object in C++ for writing data to files.
- fstream
- A file stream object in C++ that can read and write data to files.
- Buffered Input/Output
- The process of storing data in a small area of memory (buffer) before sending it in larger chunks for efficiency.
- Unbuffered Input/Output
- The process of sending data directly without buffering, resulting in immediate processing but possibly less efficiency.
- Arduino
- A microcontroller that can operate in both buffered and unbuffered modes for processing input or output data.