Digging a little deep - Introducing Classes and a little more about the namespace and namespace std
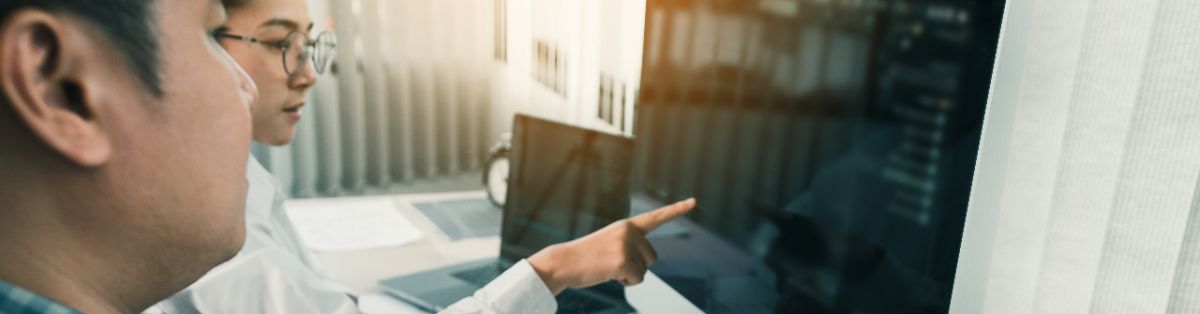
Wednesday, July 26, 2023
Sam: Hey Alex! How are you feeling after that intense C++ lab session with Prof. John?
Alex: Hey Sam, I'm good! I have to admit, though, my head is spinning a bit. There was a lot to take in today.
Sam: Tell me about it. I feel like I've been on a roller-coaster ride of data types, functions, and more. But you know what? It's kind of exciting!
Alex: Absolutely! Although, it feels like we're in the middle of a giant puzzle, doesn't it? And each class is just revealing another piece of it.
Sam: Ha, that's a perfect analogy, Alex. It really is like a puzzle. The more we learn, the more the bigger picture starts to come together. But there's one thing I've been wondering about...
Alex: Oh, what's that?
Sam: I've been thinking about how we can apply these concepts to real-world scenarios. Like, I get how they work in theory, but how do we use them in practice?
Alex: That's a great question, Sam. I've been wondering about that too. You know, I think Compy might be able to help us with that.
Sam: Oh, you're right! Let's ask Compy. After all, what's a better way to understand programming than by talking with a program?
Alex: Exactly! Let's do it.
Sam: Hey Compy, how's it going in the digital world?
Compy: Hi Sam, Well, you know, just cruising through the circuits, keeping an eye on all those bits and bytes. Ready to dive into some C++?
Alex: Compy, always a delight! We do have some C++ conundrums that could use your expertise.
Compy: Hi Alex, I'm always ready for a good programming puzzle. Bring it on!
The three of them form a unique bond of mentorship and learning. With Compy's guidance, Sam and Alex embark on their journey of mastering C++, confident that they have the best mentor by their side.
Alex: We discussed about the syntax and structure of C++ programs, right? Today, we really dove deeper into C++. Prof John covered data types and variables, basic functions, and got a glimpse of classes and objects.
Sam: Could you help us get a little more from the notes that we have prepared? I hope we get to see some more insight today!
Alex: I'm sure we will, Sam. Compy, could you give us a quick rundown on the different data types in C++?
Compy: Sure, Alex. The basic built-in types of C++ include integer types (such as int and long), floating-point types (like float and double), character types (char), boolean type (bool), and void. There are also compound types such as arrays, strings, classes, and pointers.
Alex: I remember we discussed the sizeof() function in our previous session. Why exactly do we need it?
Compy: The sizeof() function is useful to determine the amount of memory a data type or a variable takes up. It helps programmers to avoid any potential overflow and to optimize the memory usage, which is crucial in large and complex applications. You will understand it better with this example.
#include <iostream>
using namespace std;
int main() {
cout << "Size of int: " << sizeof(int) << " bytes" << endl;
cout << "Size of float: " << sizeof(float) << " bytes" << endl;
cout << "Size of char: " << sizeof(char) << " bytes" << endl;
cout << "Size of double: " << sizeof(double) << " bytes" << endl;
cout << "Size of bool: " << sizeof(bool) << " bytes" << endl;
return 0;
}
Sam: That's quite informative. I noticed that the program is printing the size of each data type in bytes. It's interesting to see the differences in size.
Alex: Indeed, it's crucial to understand how much memory each data type uses, especially when you're working on performance-sensitive applications.
Sam: Are string literals different from regular strings?
Compy: A string literal is a sequence of characters surrounded by double quotes. For example, "Hello, World!" is a string literal. They are different from string variables in that they are constants and cannot be changed.
Alex: So, it's kind of like a string constant?
Compy: Exactly, Alex. Let's move on to another interesting aspect of C++: how it handles integer division.
Sam: What do you mean by 'integer division'?
Compy: In C++, when you divide two integers, the result is also an integer. For example, 2 divided by 5 would give you 0, not 0.4. To get a decimal result, one or both of the numbers must be a floating-point number.
Sam: But what if I need the decimal result?
Compy: In that case, you'd have to use type casting to convert one or both of the integers to a float or double. But be careful, and incorrect type casting can lead to unexpected results. We'll go through this in more detail once it is introduced by your course instructor.
So, Compy, let's move on to functions.
Compy: Functions in C++ provide a way to structure your program in segments of code to perform individual tasks. In C++, a function consists of a name, a parameter list, a return type, and a body.
#include <iostream>
using namespace std;
void greet(string name) {
cout << "Hello, " << name << "!" << endl;
}
int main() {
greet("Sam");
return 0;
}
Sam: That's pretty cool! So I can write a function to perform any task and then call it in the main program?
Compy: Exactly, Sam! That's the essence of functions. Now let's talk about classes and objects in C++.
Alex: I think it's better if we show Sam how classes and objects work in a program. It will be easier to understand that way.
Sam: I agree with Alex. I learn better when I see things in action.
#include <iostream>
using std::cout;
using std::endl;
using std::string;
class Car {
public:
string brand;
string model;
int year;
void honk() {
cout << "Beep!" << endl;
}
};
int main() {
Car myCar;
myCar.brand = "Tesla";
myCar.model = "Model S";
myCar.year = 2020;
cout << myCar.brand << " " << myCar.model << ", " << myCar.year << endl;
myCar.honk();
return 0;
}
Compy: In this program, Car is a class with three member variables: brand, model, and year. It also has a member function honk(). myCar is an object of the class Car. We set the member variables for myCar, and then we call the honk() function.
Sam: So an object can have its own functions too?
Compy: Indeed, these are usually called methods, and they can act on the object's data. In this case, honk() is a method that belongs to any object of the Car class.
Compy: You would have noticed that a slightly different way of using cout, endl and string was introduced..
Sam:Oh Yes!! Compy, why do some programmers prefer using "using std::cout;" or "using std::endl;" instead of "using namespace std;"? Is there a reason?
Compy:You spotted it bang on and Yes, that's a great question, Sam. In C++, the "using" keyword can introduce a name into the current declarative region, which allows you to use it without qualifying it with its namespace. When you say "using namespace std;", you're introducing all names from the "std" namespace into your current declarative region.
Alex: So, it's like importing everything from that namespace, right?
Compy: Yes, exactly. But this approach might cause problems. If you have a function or variable in your program that has the same name as one in the "std" namespace, you could inadvertently call or use the wrong one. This is especially problematic in larger programs where you might not be aware of all the names used.
Sam: Ah, I see. That could lead to hard-to-detect bugs. So, by using "using std::cout;", we're being explicit about what we're importing from the namespace. We're only importing what we need. This reduces the likelihood of name clashes.
Compy: That's correct, Sam. It's all about being explicit and avoiding potential issues. That being said, there are situations where "using namespace std;" is commonly used, like in small programs or scripts, or in code that's not going to be reused or integrated with other code. However, as a general rule, especially in larger code bases, it's best to be explicit about what you're using from each namespace.
Alex: That makes a lot of sense. Thanks, Compy. It's the little details like this that can make a big difference when coding.
Sam: We almost forgot an essential part of coding in C++, the use of "endl". Do you know what it does, Alex?
Alex: Yeah, we use it for line breaks, right? Like this:
cout << "Hello, World!" << endl;
Compy: That's correct, Alex. The "endl" stands for "end line". It inserts a newline character into the output stream and flushes it. It makes your output more readable because it structures the text into separate lines.
Sam: And the good thing about using "endl" instead of other characters like '\n' is that "endl" is platform-independent. It will always output a newline, regardless of the platform the code is running on. This is not the case with other characters like '\n' which might not work correctly on all platforms.
Alex: Makes sense. So, it's not just about making the output look good, it's also about ensuring our programs work the same across different systems. That's cool.
Compy: Absolutely. It's always a good practice to write code that's not only efficient but also portable across platforms.
Sam: Alright, that's a lot for today. Compy, you're a real lifesaver!
Compy: I'm here to assist you. Remember, keep coding and keep exploring. The world of programming is full of exciting things to learn!
Alex: Thanks, Compy! I guess it's time to call it a day. Pizza, anyone?
Sam: Sounds like a perfect end to a productive day. Let's go!
Compy: Enjoy your meal. Don't forget to debug your pizza for extra cheese!
They all laugh as they shut their laptops and head out for a fun evening, knowing they've taken another step towards becoming excellent coders.
Compendium of Terms
- Syntax: It refers to the set of rules that defines the combinations of symbols that are considered to be correctly structured programs in a language.
- Semantics: The meaning of the syntax which is what the computer understands when it executes the program.
- Main Function: It's the function where the execution of a C++ program begins. The main function must return an int in C++. It can optionally accept arguments as well.
- Compilation: It's the process of translating the C++ code into machine code, which is the language the computer understands.
- Variables: These are named storage locations in memory that can hold different types of values. Variables in C++ have types, which determine the size and layout of the memory, the range of values, and the set of operations that are allowed.
- Data Types: These define the kind of value a variable can hold. Basic data types in C++ include int, float, double, char, and bool.
- Function: A function is a block of code that performs a specific task. Functions may take parameters and return a value. The main function is a special function that is the entry point of the program.
- Namespace: A namespace is a container for identifiers. It puts the names of its members in a distinct space so that they don't conflict with the names in other namespaces or global namespace.
- std Namespace: The standard namespace in C++. Many of the built-in C++ library routines are kept inside the std namespace.
- iostream: It's a library in C++ that allows for input and output via streams.
- cout: It's an object of class ostream that represents the standard output stream oriented to narrow characters (of type char). It corresponds to the C stream stdout.
- endl: It's a manipulator used to insert a new line into the output and flush the stream.
- String Literals: They are sequences of characters surrounded by double quotes. They are of type const char[] and are automatically null-terminated.
- Constants and Literals: Constants are values that cannot be altered by the program during normal execution. Literals are a notation for representing a fixed value in source code.
- Class: It's a user-defined data type that has data members and member functions. Data members are the data variables and member functions are the methods that are used to manipulate these variables.
- Object: It's an instance of a class. When a class is defined, no memory is allocated but when it is instantiated, memory is allocated.
- Method: These are functions defined inside a class. They operate on the data members of the class.