Fundamental Concepts of C++, a conversational approach
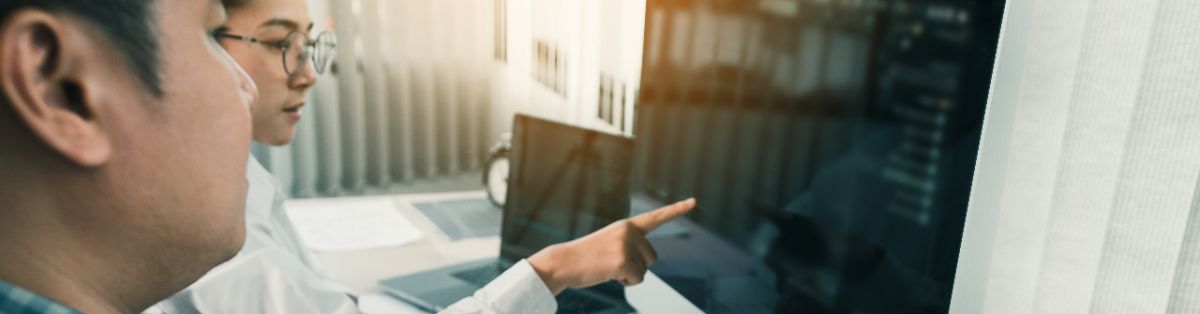
Introduction & Setting
On a sunny afternoon, Sam and Alex, two enthusiastic Civil Engineering undergrads, are putting their heads together in their computer lab, pondering the mysteries of their new Computer Science elective. With Prof. John's introductory session, the world of C++ seems intriguing yet a tad daunting. But they have a secret weapon - Compy, their trusty old computer with a cutting-edge AI personality.
Equipped with a 64-core processor, 128GB of RAM and a 4TB SSD, Compy is an absolute powerhouse. He's often fond of comparing himself to his "great-great-great-grandpa", the Apollo Guidance Computer, who helped land the first humans on the moon with just a 2KB RAM and a 1MHz processor.
Dialogue:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!";
return 0;
}
Alex: (Gazing at his computer screen in puzzlement) Sam, have you ever tried to understand these keywords in C++? It's all Greek to me.
Sam: (Leaning over to take a look) Oh, like 'int' and 'return'? Yeah, they seemed weird at first, but I think I'm getting the hang of it. Let's ask Compy!
(They boot up Compy, their talking computer.)
Compy: Hello, Sam and Alex! How can I assist you in your quest for coding knowledge today?
Alex: Compy, we were told that C++ programs are step-by-step instructions. Can you explain this more?
Compy: Absolutely, Alex. When you write a C++ program, you're essentially giving your computer a set of instructions to follow, much like a recipe. Each line of code is an instruction or step, which is executed in the order they're written. This sequence of operations is fundamental to how all programming languages work.
Sam: That's really interesting. So, it's like we're the chefs, and the computer is our assistant-chef?
Compy: Exactly, Sam! Now you're cooking! However, always remember that the computer will only do what you tell it to do, no more and no less.
Alex: Compy, what's the deal with these keywords and identifiers in C++?
Compy: Well, Alex, keywords are predefined words in C++ that have special meanings to the compiler. They're used to perform internal operations. Identifiers, on the other hand, are the names given to elements such as variables and functions.
Sam: Right, like 'int' for integers and 'return' for ending a function. So what are these tokens in C++?
Compy: Very observant, Sam! A token is the smallest element of a program that is meaningful to the compiler. It could be a keyword, an identifier, a constant, a string literal, or a symbol.
Alex: And what about these symbols like ';', '#', and the different brackets?
Compy: Ah, symbols! Each has its role. The ';' symbol indicates the end of a statement, '#' is used in preprocessor directives, and the different brackets, '()', '{}', '[]', are used in function calls, scope blocks, and array subscripting respectively.
Sam: And why does every program have this 'using namespace std' thing?
Compy: Well, 'std' is an abbreviation for standard. When we use 'using namespace std', we are telling the compiler to assume we are using the standard namespace, which is a container where identifiers, keywords and libraries reside. It helps us avoid naming conflicts in larger programs. It's a bit like how you wouldn't want two students named Alex in the same group project!
Alex: (Laughs) Yeah, that'd be confusing. Oh, and Compy, why does the 'main' function sometimes return 0, sometimes 1, and sometimes nothing?
Compy: Good question, Alex! The 'main' function's return type can be void, int, or in some cases, auto in modern C++. If it's 'int', it usually returns 0 at the end, indicating that the program has run successfully. Other values are typically used to represent different error conditions.
Sam: That's cool. Now, I remember Prof. John talking about UTF-8 and some data streams like cin, cout, and cerr. Just have a look at the following code and could you please elaborate?
#include <iostream>
using namespace std;
int main() {
float radius, area;
cout << "Enter the radius of the circle: ";
cin >> radius;
area = 3.14 * radius * radius;
cout << "Area of the circle = " << area;
return 0;
}
Compy: Certainly, Sam! UTF-8 is a universal character encoding standard that covers almost all the characters and symbols in the world. As for the data streams, 'cin' is used for input, 'cout' for output, and 'cerr' for outputting error messages.
Alex: And we've been saving our C++ code with a .cpp extension. Why is that so?
Compy: .cpp is the standard extension for C++ source files. After you write your code, it gets compiled into a different format that the machine can execute directly. That's the difference between source files and compiled files.
Alex: It's a bit overwhelming with all these different parts, but it's starting to make sense. There's one thing I've noticed, though - all of the C++ source files we've worked on have the extension .cpp. Is there a reason for this?
Compy: Good observation, Alex! The .cpp extension stands for C++ and is used to denote source files written in the C++ language. This helps the compiler recognise the file and compile it correctly.
Sam: So, it's like how we use .docx for Word documents?
Compy: Precisely, Sam! It's all about helping the right program recognise and work with the file. Now, remember that once the .cpp file is compiled, it's turned into a binary file that your computer can directly execute. These compiled files often have different extensions, like .exe on Windows.
Sam: So we write step-by-step instructions in the source file, and the computer follows them?
Compy: Exactly, Sam! That's programming in a nutshell. But remember, even if the hardware has evolved from the likes of my great-great-great-grandpa, the essence of programming remains the same. And talking about hardware evolution, did you know that the line endings we use, CR, LF, and CRLF, have an interesting backstory?
Alex: No way! What's the story, Compy?
Compy: You see, CR stands for Carriage Return, and LF stands for Line Feed. These terms date back to the typewriter days. A carriage return, or CR, meant returning to the start of the line after typing. Line feed, or LF, meant moving down to the next line.
Sam: That's so cool. But why do we have different line endings now?
Compy: Well, different systems adopted different notations. Unix systems use LF to mark the end of a line, while Macintosh used CR. Windows decided to use both, hence CRLF. This can sometimes cause compatibility issues when transferring text files between systems.
Alex: Fascinating! But back to our program, how does the computer know what 'cout' and 'cin' are? Are they built-in commands?
Compy: Not exactly, Alex. They're part of the std namespace which stands for standard. Namespaces are a way in C++ to group identified names (like cin and cout) under a specific name, preventing naming collisions. That's why you often see 'using namespace std' at the start of programs.
Sam: Wow, Compy, you've really made this so much clearer.
Compy: I'm glad I could help! Remember, C++ is case-sensitive, so always pay attention to your capitalisation. 'Cout' and 'cout' would be considered different identifiers!
Alex: I think I'm starting to get the hang of this. But we also learned about something called memory management in C++, right?
Compy: Yes, indeed! In C++, you have direct control over both dynamically and statically allocated memory. This is very powerful, but also means you have to make sure to manually manage the allocation and deallocation of memory to prevent leaks. It's a big topic, but don't worry, you'll get the hang of it!
Sam: I can't wait! Thanks, Compy.
Compy: Anytime, Sam! I'm here to help you both navigate the exciting world of C++. Don't forget to practice, and always refer to quality resources online and compilers for hands-on experience. And remember, just like Apollo 11 reached the moon with my ancestor's help, you too can achieve great things with programming. After all, the future of civil engineering is digital!
Compendium of Terms
- C++ Programming Language: It's a general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language, or "C with Classes".
- Keywords and Identifiers: These are the basic building blocks of a C++ program. Keywords are reserved words that have special meaning in C++, while identifiers are names given to different entities such as variables and functions.
- Tokens in C++: These are the smallest individual units in a program, including keywords, identifiers, literals, symbols, etc.
- Significance of ';', '#', different brackets and ' ': The semicolon (;) denotes the end of a statement, the hash symbol (#) is used in preprocessor directives, different types of brackets are used for different purposes (e.g., function calls, code blocks), and whitespace (' ') is used to separate tokens.
- UTF-8: This is a character encoding used to represent text in computers, databases, and communication systems.
- Data Streams (cin, cout, cerr): These are objects used for input/output operations. 'cin' is used for input, 'cout' for output, and 'cerr' for error output.
- Line Endings (CR, CRLF, LF): Different systems use different symbols to represent the end of a line in a text file. CR (Carriage Return) is used by Mac OS, CRLF (Carriage Return Line Feed) by Windows, and LF (Line Feed) by Unix/Linux.
- The Namespace std: This namespace includes features of the C++ Standard Library, such as cout and cin. By using the 'using namespace std' statement, we can use these features without prepending 'std::'.
- Different Return Types of Main: The 'main' function can return different values to indicate how the program terminated, typically 0 for successful completion.
- Characters with Escape Sequences ('\n', '\r', '\t'): These are used to represent newline, carriage return, and tab respectively.
- C++ is Case Sensitive: The language differentiates between uppercase and lowercase letters.
- C++ Source Files vs Compiled Files: Source files contain the original code written by the programmer, while compiled files contain the machine code generated by the compiler.
- C++ Requires an Extension of .cpp: This is the standard file extension for C++ source files.
- Memory Management in C++: This involves allocating and deallocating memory for variables and data structures during runtime.
- Programs as Step-by-Step Instructions: A C++ program consists of a series of instructions that the computer executes sequentially.